Java Lab 1– Foundation Computing
Instructions:-
Task 1: Write a nested if statement to display the following information (Run the code in the IDE and save your program as Selection1.java)
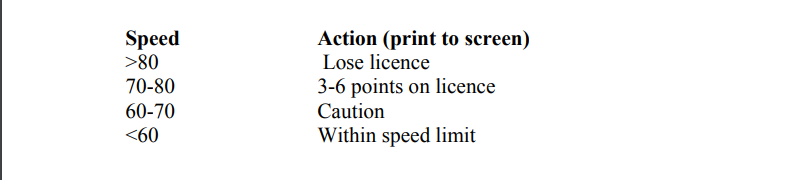
Task 2: Write a code fragment that reads in a double and outputs a sentence “Between 0 and 5.5” or “Not between 0 and 5.5”, depending on the value read in.
(Run the code in the IDE and save your program as Selection2.java)
Task 3: Write a code fragment to read in 3 integers and print out the largest. (Run Run the code in the IDE and save your program as Selection3.java)
Task 4: To create a histogram for a set of numbers in the range 1 to 50, we have to find out what bin a number will be put into.
(Run Run the code in the IDE and save your program as Selection4.java)
The bins will be spaced as follows
Bin 1: 1-10
Bin 2: 11-20
Bin 3: 21-30
Bin 4: 31-40
Bin 5: 41-50
Write a code fragment that asks the user to enter a number between 1 and 50 and then outputs the bin number in which it would be put.
Task 5: Write a switch statement that prints a message
(Run Run the code in the IDE and save your program as Selection5.java)
By entering a mark (from 0-100), a message will be displayed on the screen and indicates whether grade (a character) the mark is – ‘A’,’B’ or ‘C’, in which case print “You Have Passed”, or ‘D’, ‘E’ in which case print “You have failed: You must resit the exam”, or ‘F’, then print out “You have failed: You cannot resit the exam”. Otherwise print out “Unknown Grade”
Grade A: 70-100 Grade
B: 60-70
Grade C: 50-60
Grade D: 40-50
Grade E: 30-40
Grade F: below 30
Task 6: Repeat exercise 5 using a nested if statement instead of a switch statement
(Run Run the code in the IDE and save your program as Selection6.java)
Task 7: What is the output from the following code?
int max;
int min = 10;
max = 17 – 4 / 10;
max = max + 6;
min = max – min;
System.out.println(max * 2);
System.out.println(max + min);
System.out.println(max);
System.out.println(min);
Task 8: Write a complete java program to ask the user enter the values for three integer variables for the following expressions. After the following code? (What is the code really doing?)
int a = 3;
int b = 7;
int c = 9;
a = a * b * c;
b = a / b / c;
c = a / b / c;
a = a / b / c;
Task 9: Write a complete java program to ask the user the following questions:
- How tall are you in inches as integer datatype?
- Are you tall enough to ride the roller coaster?
- The variable isTallEnought as boolean datatype.
- Scanner or Java option pane for input datatype
- The condition for isTallEnough >=37.
Task 10: Write a program to calculate the cost of delivery for an order from a local supermarket. The current rates for different items are shown below (Save your program as AmazonDelivery.java) (JOptionPane)!!!

“per delivery charge” is a single charge per order. It is always charged at the most expensive rate for any of the items ordered.
For example:
1 Book – would cost a total of £5.42 (1 x £3.26 + £2.16) ·
2 CDs + 1 Game – would cost a total of £16.97 (2 x £1.39 + 1 x £12.60 + £1.59) – the delivery charge is £1.59 as the delivery charge of a game is greater than that of a CD. The requirements of the program are:
a) Ask the user to enter how many of each item they are buying;
b) Make sure that the input is valid, i.e. an integer greater than zero.
c) Calculate the cost of delivery.
d) Print out a neatly formatted break down of the delivery cost for example:
Solution
Foundation Computing -Java Lab 1 Assignment Help.
Number 1
public
class speedlimit{
//I want to
create a nested if statement displaying the speed limit
public static void main(String[] args) {
printMessage(74);
}
public static void printMessage(int i)
{
if (i > 80)
{
System.out.println("Lose
licence");
}
else if(i
> 70)
{
System.out.println("3-6 points on
licence");
}
else if(i
> 60)
{
System.out.println("Caution");
}else
{
System.out.println("Within speed
limit");
}
}
}
Number 2
public class selection{
//a code fragment that reads in a double and outputs a specified sentence
public static void main(String[] args){
opinionDoubleNum(3.6);
}
public static void opinionDoubleNum(double i)
{
if(i >= 0 && i <= 5.5)
{
System.out.println(“Between 0 and 5.5”);
}
else
{
System.out.println(“Not between 0 and 5.5”);
}
}
}
Number 3
public class integers{
/*a code that reads three integers.
The code prints out the largest.
*/
public static void main(String[] args) {
System.out.println(getMax01(3,21,7));
System.out.println(getMax02(3,21,7));
}
public static int getMax01(int q, int p, int r){
return q > p ? q > r? q : r : p > r ? p : r;
}
public static int getMax02(int q, int p, int r){
if(q > p){
if(q > r)
return q;
return r;
}else{
if(p > r)
return p;
return r;
}
}
}
Number 4
package histogram;
import java.io.*;
//import java.util.Scanner;
/*Creates a histogram.
A range of numbers between one and fifty.
Finds out what bin a number lies.
*/
public class histogram {
public static void main(String[] args) {
printBin();
}
private static void println() {
// TODO Auto-generated method stub
}
public static void printBin() {
java.util.Scanner sc = new java.util.Scanner(System.in);
int temp = sc.nextInt() / 10;
switch (temp) {
case 0:
System.out.println(“Bin 1”);
break;
case 1:
System.out.println(“Bin 2”);
break;
case 2:
System.out.println(“Bin 3”);
break;
case 3:
System.out.println(“Bin 4”);
break;
case 4:
System.out.println(“Bin 5”);
break;
default:
System.out.println(“number lies outside 1 and 50”);
break;
}
}
}
Number 5
public class grading{
public static void main(String[] args) {
printMessage(68);
}
/*a grading code.
Gives conditions for ‘fail’grades.
*/
public static void printMessage(int i) {
int temp = i / 10;
switch (temp) {
case 10:
case 9:
case 8:
case 7:
//cases 7 to ten lie above 70; hence pass.
System.out.println(“Grade A :You Have Passed”);
break;
case 6:
System.out.println(“Grade B :You Have Passed”);
break;
case 5:
System.out.println(“Grade C :You Have Passed”);
break;
case 4:
System.out.println(“Grade D :You Have failed,You must resit the exam”);
break;
case 3:
System.out.println(“Grade E :You Have failed,You must resit the exam”);
break;
case 2:
case 1:
case 0:
System.out.println(“Grade F :You Have failed,You must resit the exam”);
break;
default:
System.out.println(“Unknown Grade”);
break;
}
}
}
Number 6
public class selection{
public static void main(String[] args) {
printMessage(68);
//finds the expressions value and give conditions for ‘fail’ grade
}
public static void printMessage(int i) {
if(i > 100) {
System.out.println(“Unknown Grade”);
}else if(i >= 70){
System.out.println(“Grade A :You Have Passed”);
}else if(i >= 60){
System.out.println(“Grade B :You Have Passed”);
}else if(i >= 50){
System.out.println(“Grade C :You Have Passed”);
}else if(i >= 40){
System.out.println(“Grade D :You Have failed,You must resit the exam”);
}else if(i >= 30){
System.out.println(“Grade E :You Have failed,You must resit the exam”);
}else{
System.out.println(“Grade F :You Have failed,You cannot resit the exam”);
}
}
}
Number 7
package codeoutput;
public class codeoutput {
public static void main ( String[] args) {
int max;
int min =10;
max=17-4/10;
max=max+6;
min=max-min;
System.out.println (max*2);
System.out.println (max+min);
System.out.println (max);
System.out.println (min);
//gives the output displayed below
}
}
Output
46
36
23
13
Number 8
package Mathcalc;
import java.io.*;
//import java.util.Scanner;
public class Mathcalc {
public static void main(String[] args) {
java.util.Scanner sc = new java.util.Scanner(System.in);
System.out.println(“Enter integer value for a:”);
float a = sc.nextInt();
System.out.println(“Enter integer value for b:”);
float b = sc.nextInt();
System.out.println(“Enter integer value for c:”);
float c = sc.nextInt();
a=a*b*c;
System.out.println(a);
b=a/b/c;
System.out.println(b);
c=a/b/c;
System.out.println(c);
a=a/b/c;
System.out.println(a);
}
}
Number 9
package rollercoaster;
import java.io.*;
//import java.util.Scanner;
public class rollercoaster {
public static void main(String[] args) {
java.util.Scanner sc = new java.util.Scanner(System.in);
System.out.println(“How tall are you:”);
int height =sc.nextInt();
Boolean isTallEnough;
if(height>=37){
isTallEnough=true;
System.out.println(“You are tall enough to ride the roller coaster!”);
}else {
isTallEnough =false;
System.out.println(“You are not tall enough to ride the roller coaster!”);
}
}
}
Number 10
package Amazon;
import java.util.*;
//import java.util.Scanner;
public class Amazon{
//calculates the cost of delivery for each order.
public static void main(String[] args) {
Item book = new Item(“Book”, 3.26,2.16);
Item cd = new Item(“CD”,1.39,1.16);
Item dvd = new Item(“DVD”,3.49,1.16);
Item game = new Item(“Game”,12.60,1.59);
Scanner sc = new Scanner(System.in);
System.out.println(“2”);
int b = sc.nextInt();
while(b < 0){
System.out.println(“Enter a non-negative number”);
b = sc.nextInt();
}
System.out.println(“4”);
int c = sc.nextInt();
while(c < 0){
System.out.println(“Enter a non-negative number”);
c = sc.nextInt();
}
System.out.println(“0”);
int d = sc.nextInt();
while(d < 0){
System.out.println(“Enter a non-negative number”);
d = sc.nextInt();
}
System.out.println(“1”);
int g = sc.nextInt();
while(g < 0){
System.out.println(“Enter a non-negative number”);
g = sc.nextInt();
}
double higEx = 0;
if(b > 0) higEx = Math.max(higEx, book.getExPrice());
if(c > 0) higEx = Math.max(higEx, cd.getExPrice());
if(d > 0) higEx = Math.max(higEx, dvd.getExPrice());
if(g > 0) higEx = Math.max(higEx, game.getExPrice());
System.out.println(“Item\tNumber\tCost\tTotal”);
System.out.println(book.getName()+”\t”+b+”\t”+book.getPrice()+”\t”+b*book.getPrice());
System.out.println(cd.getName()+”\t”+c+”\t”+cd.getPrice()+”\t”+c*cd.getPrice());
System.out.println(dvd.getName()+”\t”+d+”\t”+dvd.getPrice()+”\t”+d*dvd.getPrice());
System.out.println(game.getName()+”\t”+g+”\t”+game.getPrice()+”\t”+g*game.getPrice());
System.out.println(“Delivery Charge\t” + higEx +”\t” + higEx);
System.out.println(“Total\t\t\t” + (b*book.getPrice()+c*cd.getPrice()+d*dvd.getPrice()+g*game.getPrice()+higEx));
}
}
class Item{
private String name ;
private double price;
private double exPrice;
public double getExPrice() {
return exPrice;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
public Item(String name, double price, double exPrice){
this.name = name;
this.price = price;
this.exPrice = exPrice;
}
}